Interact with the vRealize Automation IaaS objects on vRealize Orchestrator
As described on another blog post, vRA IaaS objects can be gathered using the model manager.
This article will show you how to interact with this kind of object using vRealize Orchestrator
.
On this first sample, we are trying to get a virtual machine named TESTVM02
on the model manager.
The VirtualMachine object has a property called VirtualMachineName
that we can used to filter the virtual machine by its name.
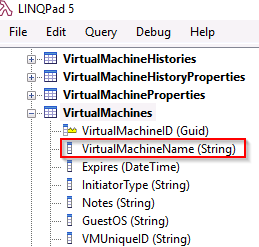
Image
Here is the javascript code in order to get this virtual machine machine. It will return a object of type VCAC:Entity.
1// Inputs : vcacHost : vCAC:vCACHOST
2
3// Define the virtual machine name to search
4var vmName = 'TESTVM02';
5
6// Use the management model
7var modelName = 'ManagementModelEntities.svc';
8// Get the virtual machines object
9var entitySetName = 'VirtualMachines';
10// Filter the result by the property VirtualMachineName
11var filter = "VirtualMachineName eq '"+vmName+"'";
12var orderBy = null;
13var top = null;
14var skip = 0;
15var headers = null;
16var select = null;
17
18// Query the model manager
19var vms = vCACEntityManager.readModelEntitiesBySystemQuery(vcacHost.id, modelName, entitySetName, filter, orderBy, select, top, skip, headers);
20// Check if the virtual machine has been found
21if(vms == null || vms == ''){
22 var message = 'There is no virtual machine named '+vmName;
23 System.warn(message);
24}
25else{
26 var message = 'Virtual machine '+vmName+' found';
27 System.log(message);
28}
Workflow in action :
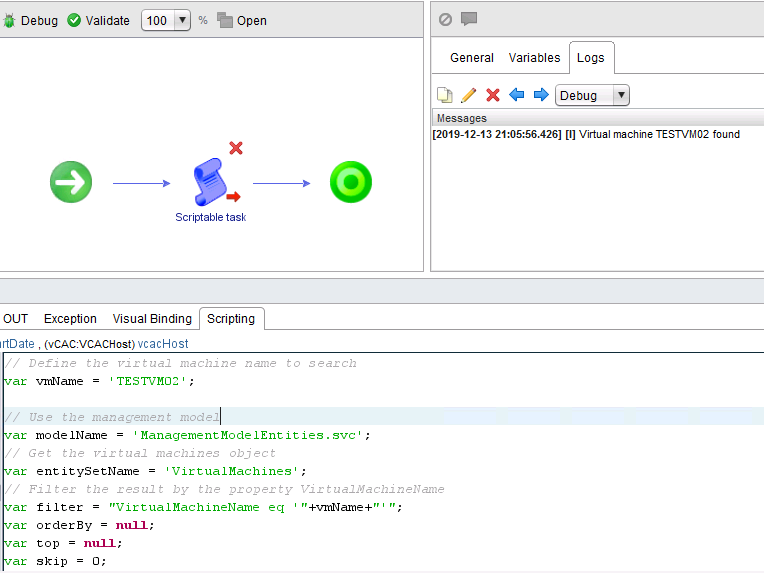
Image
Great ! Once the virtual machine captured, we can access to all its properties.
For instance, we need to know the vCPU and RAM configured on the virtual machine.
The request is simple, we'll use the method get_property
on the object VCAC:Entity
You just need to get the corresponding properties names:Image
1// Get the first virtual machine on the result (There is only one as we used the filter)
2var vm = vms[0];
3var vmVcpu = vm.getProperty('VMCPUs');
4var vmRam = vm.getProperty('VMTotalMemoryMB');
5System.log('The virtual machine '+vmName+' is configured '+vmVcpu+' vCPU and '+vmRam+' RAM');
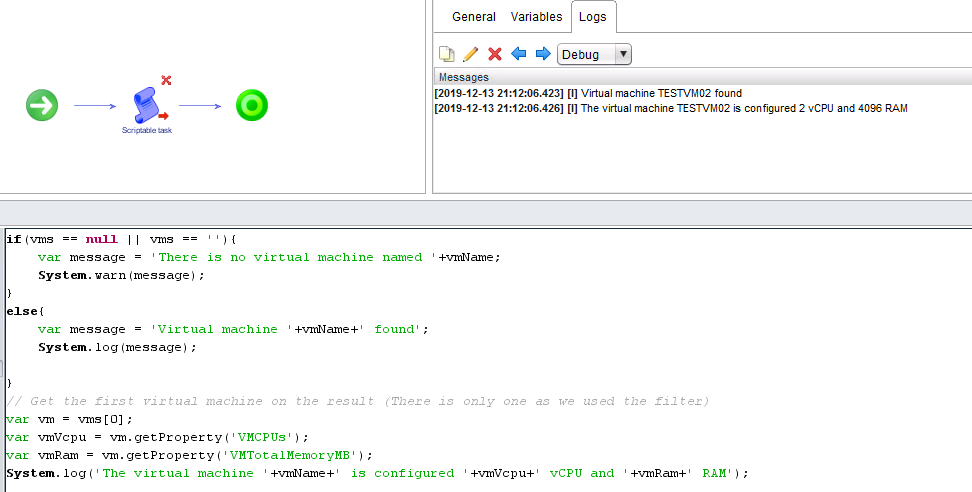
Image
Everything is working well, but now we also want to display the virtual machine hard-disks.
There is a property name called VMDiskHardware
but not directly accessible because this is a child object of VirtualMachine
.
Using LINQPAD, we can access to this property by using the method expand
1VirtualMachines.Expand("VMDiskHardware").Where (v => v.VirtualMachineName == "TESTVM02")
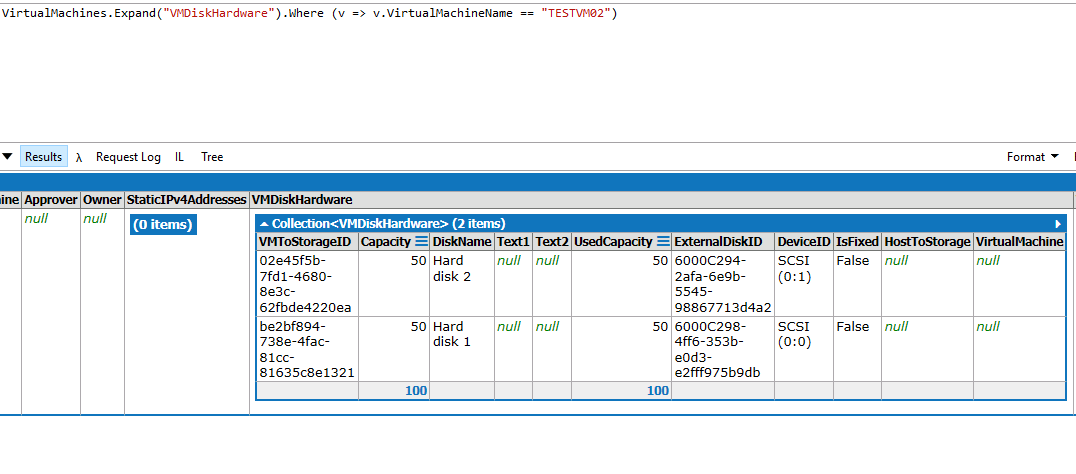
Image
With vRO, we have to use the the method get_link
of the corresponding VCAC:Entity
This method has 2 parameters :
- The first one is your IaaS manager (
vCAC:vCACHost
object on vRO ) - The second is the link name ( the child object name ).
1var vmDisks = vm.getLink(vcacHost, 'VMDiskHardware');
2for each(var vmDisk in vmDisks){
3 diskName = vmDisk.getProperty('DiskName');
4 diskSize = vmDisk.getProperty('Capacity');
5 System.log(diskName+' is configured with '+diskSize+' GB');
6
7}
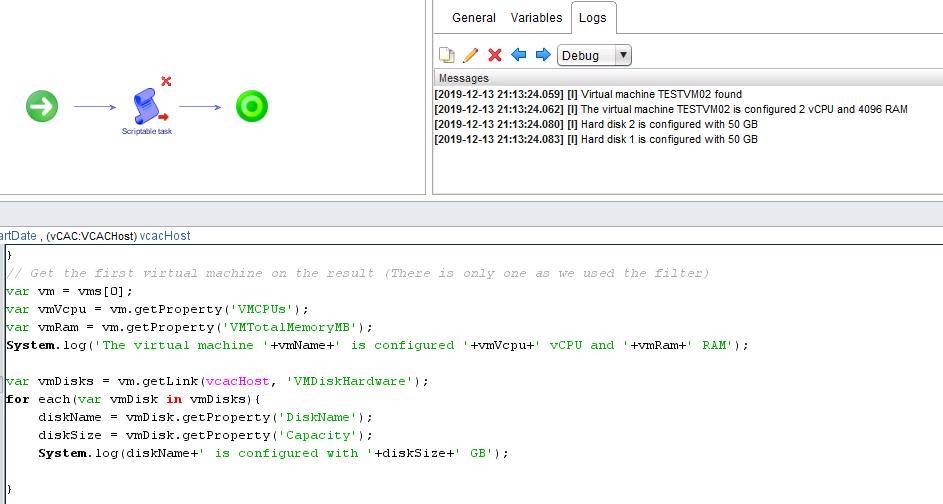
Image
Perfect ! We can now request the IaaS database in order to get a lot of usefull information to extend the use on vRealize Automation.
To end this blog post, here is another sample to get the VirtualMachine created after a defined date :
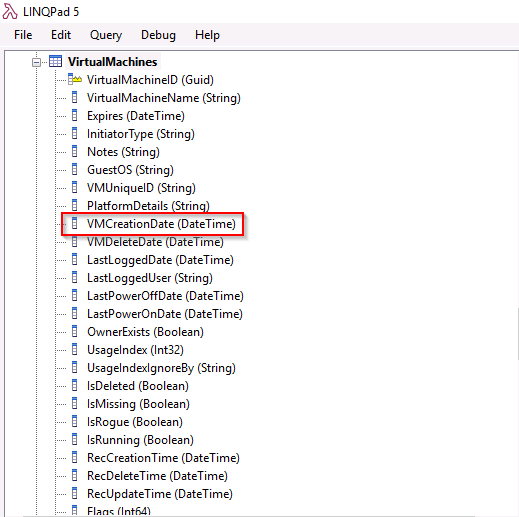
Image
1// Inputs : startDate : Date; vcacHost : vCAC:vCACHOST
2
3startDateFormatted = System.formatDate(startDate, "yyyy-MM-dd")+'T00:00:00';
4
5//Define the model name
6var modelName = 'ManagementModelEntities.svc';
7//Get the virtual machines
8var entitySetName = 'VirtualMachines';
9
10//Get all the vcac vm created after the defined date
11var filter = "VMCreationDate ge datetime'"+startDateFormatted+"'";
12var orderBy = null;
13var top = null;
14var skip = 0;
15var headers = null;
16var select = null;
17
18//Query the database
19var vms = vCACEntityManager.readModelEntitiesBySystemQuery(vcacHost.id, modelName, entitySetName, filter, orderBy, select, top, skip, headers);
20if(vms == null || vms == ''){
21 var message = 'There is no created virtual machine after '+startDateFormatted;
22 System.warn(message);
23}
24else{
25 var message = 'There is '+vms.length+' created virtual machine after '+startDateFormatted
26 System.log(message);
27}
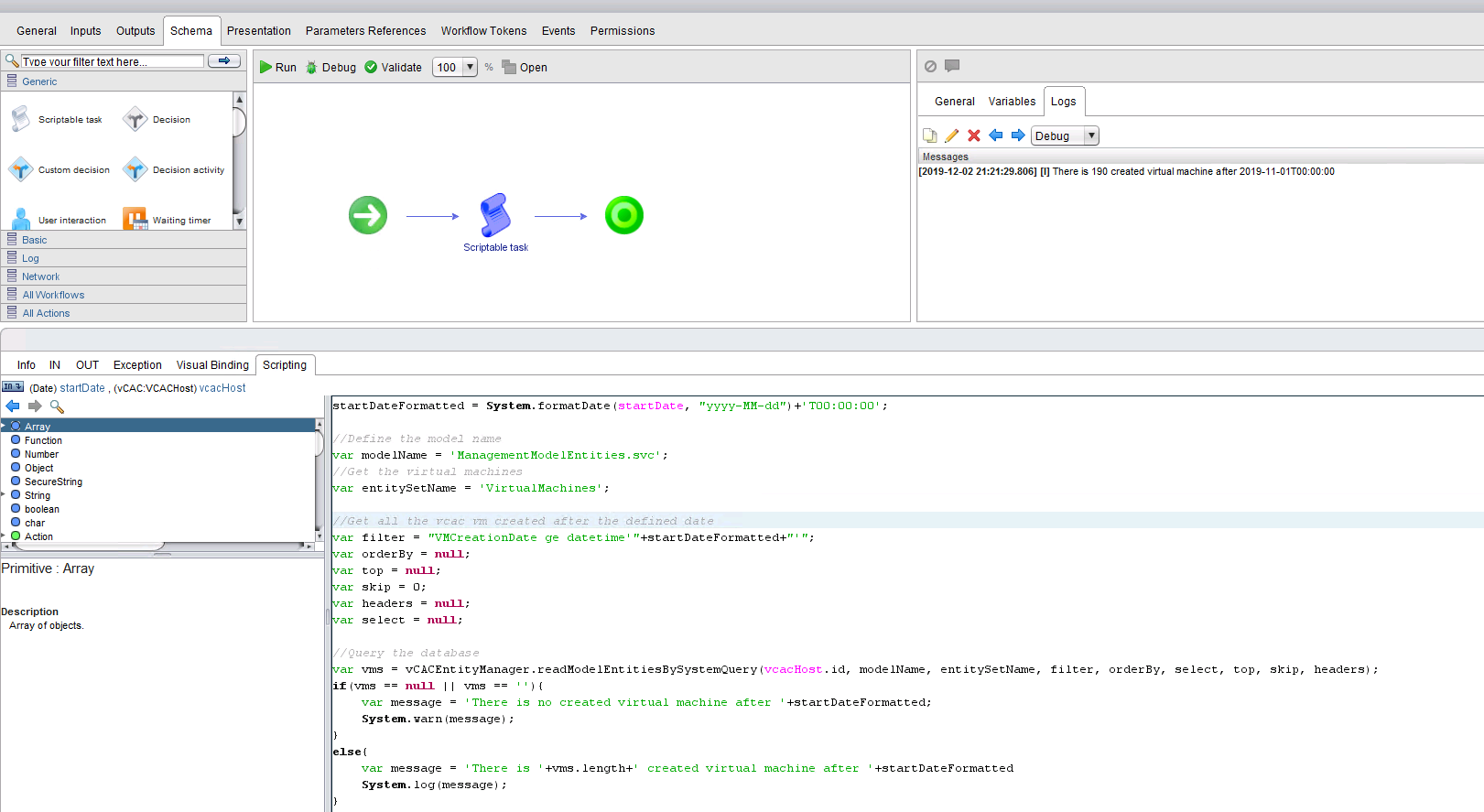
Image
You are now ready the use the IaaS object directly in vRO ;)